Percentage-wise display of RGB color from red through yellow to green
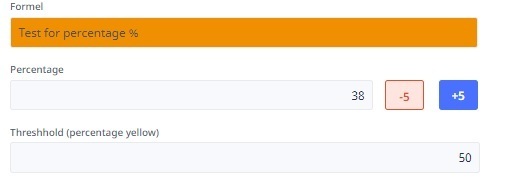
I am thinking of a nice way of displaying a status in terms of color-coding that is entirely fluid.
I was thinking to go from red through yellow to green, and maybe I will define blue as the color for an actual 100% "or process step done"
I developed four global functions for this in total. One to translate hex into numbers, one to translate numbers into hex, one to define a RGB based on percentage and a final one I picked up from other Ninox users and adapted it to define a darkness level so that you can define whether to use white font color or black font color (contrast to background)
The value "perc" is a number between 0 and 100, the value "threshhold" indicates at what percentage the color should be yellow and start shifting to green
function fnconvhex(num : number) do
"This function takes a number (should be between 0 - 255) and turns it into a 2-digit hex-code (returns string)";
let hex1 := "0";
let hex2 := "0";
let part1 := floor(num / 16);
if part1 < 10 then
hex1 := text(part1)
else
switch part1 do
case 10:
(hex1 := "A")
case 11:
(hex1 := "B")
case 12:
(hex1 := "C")
case 13:
(hex1 := "D")
case 14:
(hex1 := "E")
case 15:
(hex1 := "F")
default:
void
end
end;
let part2 := num - floor(num / 16);
if part2 < 10 then
hex2 := text(part2)
else
switch part1 do
case 10:
(hex2 := "A")
case 11:
(hex2 := "B")
case 12:
(hex2 := "C")
case 13:
(hex2 := "D")
case 14:
(hex2 := "E")
case 15:
(hex2 := "F")
default:
void
end
end;
hex1 + hex2
end;
function fnperccol(perc : number,threshhold : number) do
"This function takes a percentage value (perc) and returns a 6-digit RGB hex color (#FFFFFF) from red to yellow color until (threshhold) value and then from yellow to green";
let myvar := 200;
let myhex := "";
if perc <= threshhold then
myvar := round(200 * perc / threshhold);
myhex := "#F0" + text(fnconvhex(myvar)) + "00"
else
myvar := 230 - round((perc - threshhold) / (100 - threshhold) * 200);
myhex := "#" + text(fnconvhex(myvar)) + "CC00"
end;
myhex
end;
function fnhexdarkness(myRGB : text) do
"This function analyzes a RGB hex-color code and defines a darkness value to determine whether bright or dark font is better";
let mynum1 := fnhex2num(substr(myRGB, 1, 3));
let mynum2 := fnhex2num(substr(myRGB, 3, 5));
let mynum3 := fnhex2num(substr(myRGB, 5, 7));
if 200 - (sqrt(0.299 * pow(mynum1, 2) + 0.587 * pow(mynum2, 2) + 0.114 * pow(mynum3, 2))) < 29 then "#505050" else "white"
end
end
2 replies
-
Disadvantage of this: your dashboard will be full of all sorts of color shades of red, orange, yellow and green
-
Thank you for this proposal.
You can optimize your fnconvhex function as follows (extract from this post : https://forum.ninox.com/t/h7hn568?r=q6hn5tv)
function decToHexa(value : number) do var hexa := ["0", "1", "2", "3", "4", "5", "6", "7", "8", "9", "A", "B", "C", "D", "E", "F"]; var u := value % 16; var d := floor(value / 16); item(hexa, d) + item(hexa, u) end;
Content aside
-
2
Likes
- 2 yrs agoLast active
- 2Replies
- 217Views
-
2
Following